While developing a web application you often require different environment variables to test your application. We should know the importance of .env file in Laravel, as it is used in many purposes. For example:
- Local or Development
- Production
- Staging
There may be cases, where different developers work on the same application. Therefore, they might need to set the environment differently as compared to others. So, Laravel provides you with .env file that will work across different environments.
Note: .env file should not be committed to git project.
Laravel’s default .env
file contains some common configuration values. These may differ based on whether your application runs on a production server or locally.
Laravel also provides you the .env. This is an file where you can only add placeholder keys so that different developer or environment may use varying values as per their need.
You should not add sensitive information either in .env or .env. This example file can create security issue and hackers may easily sniff private data from your repository.
Consider the diagram below:
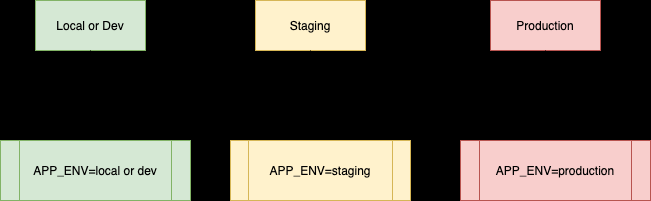
You have three different web environments to test your app. For example: dev/local, production or staging. Each of the environment uses different .env file because we cannot commit .env file. Therefore, you have to create this file manually on each environment.
Now, we have set our APP_ENV variable on each environment with varying values. Say you can run any logic when env is either local or staging. You can use the following Laravel function to check the environment.
if (App::environment('prduction')) {
// The environment is production
// Run your production env specific logic here
}
if (App::environment(['local', 'staging'])) {
// The environment is either local OR staging...
// Run your ocal OR staging env specific logic here
}
The .env file is important for many purpose. For example, to use the AWS S3 Storage bucket, it is used to add the “Access Key ID” and “Secret Access Key” variables. There are many other use cases for this file. Therefore, we should know the importance of .env file in Laravel.
You can learn here, for how to fetch specific key from .env in Laravel Controller Class.