The Controller is one main part of the Laravel MVC (Model View Controller) application structure. Let’s have a look at how the Laravel 8 controller work to use it efficiently.
Here are some examples why we use a Laravel 8 Controller Class.
- Controller makes use of the Request object and then reads the relevant data it needs
- Controller can do validation of data from an incoming Request
- Controller don’t need to implement all business logic inside it, instead you may call a service (a Controller function) that runs your business logic and returns data
- Once your service will return appropriate data you can pass this data to your view blade
Consider the diagram below:
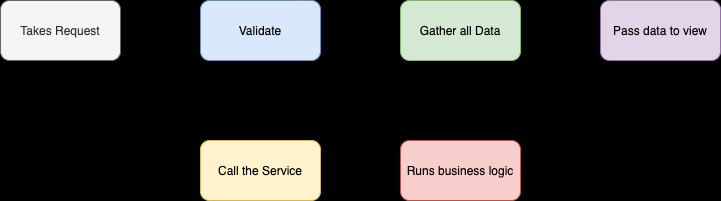
In some cases you don’t need to run business logic. In such cases pass the required data for the view to Laravel blade view directly.
Consider the simple controller example below.
<?php
namespace App\Http\Controllers;
use Illuminate\Contracts\Foundation\Application;
use App\Http\Requests\CartItemStoreRequest;
use App\Http\Service\ShoppingCartService;
use Illuminate\Contracts\View\Factory;
use Illuminate\Contracts\View\View;
use Illuminate\Http\JsonResponse;
use Illuminate\Http\Request;
class ShoppingCartController extends Controller
{
/**
* @param Request $request
* @return Application|Factory|View
*/
public function list(Request $request)
{
return view('cart');
}
/**
* @param CartItemStoreRequest $request
* @param ShoppingCartService $service
* @return JsonResponse
*/
public function store(CartItemStoreRequest $request, ShoppingCartService $service): JsonResponse
{
// Retrieve the validated input data...
$validatedData = $request->validated();
// your service handle business logic
$cartItem = $service->addItem($validatedData);
// passing data to view
return response()->json([
'item' => $cartItem->getItem()
]);
}
}
Explanation:
- The list method above looks pretty simple. We don’t need any validation or run a business logic.
- Let us go through the ShoppingCartController@store method in detail
- It first uses the validate method to confirm if the input fields are in the specified formats.
- When a request is validated you can move forward to save that data
- Now, you have a separate service which enables you to inject data in your Laravel controller using DI concept
- You may now run your business logic in that service and get data that you need
- Finally, pass this data to your view and show your data in Laravel blade view
Benefits using the above strategy
The above strategy gives you the following benefits.
- A clean looking controller
- Code is decoupled and easier unit testing
- Your service runs your business logic