The $loop
variable is used in Laravel to provide information about the current iterating loop over collections and arrays. We can access various properties and methods using the loop and do actions based on the iteration index and other details. Let’s check on how to use the $loop
variable in Laravel.
What is $loop in Laravel?
The $loop
variable is a stdClass
object that will provide access to useful metadata about the current loop. It has many useful properties as shown below.
$loop variable use cases
$loop->index
returns a 0-based current loop iteration, where 0 is the first iteration.
$loop->remaining
returns the number of iterations left over in the loop. If there are a total of 12 iterations and the current iteration is 4, it would return 8.
$loop->iteration
will return a 1-based current loop iteration, where 1 is the first iteration.
$loop->count
returns the total number of iterations or the total number of items in the array.
$loop->first
will return true for the first iteration or item in the loop or else returns false.
$loop->last
returns true for the last iteration or item in the loop or else returns false.
$loop->depth
returns the depth or nesting level of the current loop; it returns 2 if it is a loop inside a loop or 3 if it is nested at one more level.
$loop->parentIf
, the loop is nested within another @foreach loop, and the parent returns the parent’s loop.
The loops features are pretty easy to use and understand, consider the code snippet below on how to use them in a view file.
@foreach($items as $item)
@if($loop->first)
<p>Our first element of the array</p>
@endif
<p>{{ $loop->iteration . '/' . $loop->count }}</p>
@if($loop->last)
<p>Our last element of the array</p>
@endif
@endforeach
We can create a reference to the parent loop $loop
variable while we are in a nested loop. The use of depth
helps determine the nesting level of the loop. Then, you can use the parent
to get the $loop
variable of the parent. If you are nested further, you can chain the parent property again to get the $loop
variable of the parent. Consider the code snippet below for a depth of 3.
$items = [
'1' => [
'1.1' => [
'1.1.1', '1.1.2'
],
'1.2' => [
'1.2.1', '1.2.2'
]
],
'2' => [
'2.1' => [
'2.1.1', '2.1.2'
],
'2.2' => [
'2.2.1', '2.2.2'
]
]
];
Here is our code in the view file, the use of parent
helps recursively go through the full array. The code is only for a demonstration to show you that we can go up to any level of depth.
<ul>
@foreach($items as $item => $value)
<li>{{ $item }} == {{ $loop->iteration }}
<ul>
@foreach($value as $item_second => $value_second)
<li>{{ $item_second }} == {{ $loop->parent->iteration }}.{{ $loop->iteration }}
<ul>
@foreach($value_second as $value_third)
<li>{{ $value_third }} == {{ $loop->parent->parent->iteration }}.
{{ $loop->parent->iteration }}.{{ $loop->iteration }}
</li>
@endforeach
</ul>
</li>
@endforeach
</ul>
</li>
@endforeach
</ul>
In the above highlighted code section, we are just iterating on the parent to loop through and create a neat pattern.
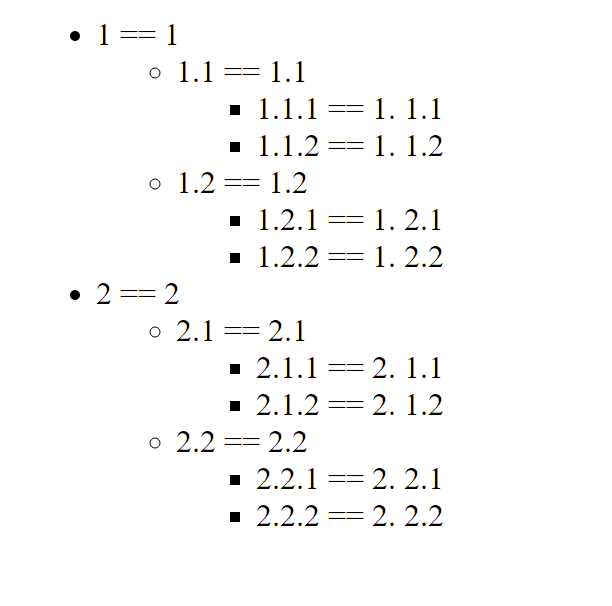