Laravel Framework allows us to use the Sail interface which will help you to create and manage your Laravel app using the docker environment. It is a command line interface that helps you manage the docker environment.
How does Laravel Sail work?
Advantage of using sail is that all your project dependencies are managed by docker. Laravel Sail enables all project dependencies to be managed well by the docker.
If your project requires multiple dependencies, for example, PHP, MySQL, Redis, or even more. Sail will install all the dependencies using a docker. This will reduce your work to add any of the dependencies explicitly to your local machine to make your project work properly.
The following diagram below illustrates how Laravel Sail works.
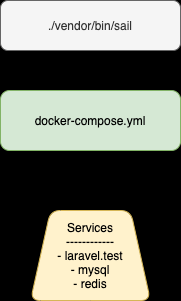
A docker-compose.yml file in your Sail project defined various services a project requires. For example, if your project needs to work with PHP, MySQL, and Redis dependencies.
Then you may see all of these dependencies under services in your docker-compose.yml file. Basically, it tells the sails containers for the mentioned dependencies should be created under services in the docker-compose.yml file.
When sails executes, it pulls all the required images from dockerhub and creates a container for each service that is defined in your docker-compose.yml file. You can even add more services that you may need based on your project.
This makes you save a huge time that you spend for local installation of php, Redis or MySQL or any other dependencies on your machine.
Installation in New Laravel Project
The following dependencies should be added as prerequisites on your mac or Linux server.
- Curl
- Docker
After adding these dependencies, you may create a new Laravel project with Sail. Type in the commands below in your terminal
# install laravel using curl
# change example-app to your project name
curl -s "https://laravel.build/example-app" | bash
# once installed run docker containers using
./vendor/bin/sail up
# to destroy running container run
./vendor/bin/sail down
Once your project is created locally you may find sail in the location below:
./vendor/bin/sail
Installation in existing Laravel project
You can also add Laravel Sail in your existing Laravel project. Execute the commands below for this.
# install composer dependency for sail
composer require laravel/sail --dev
# publish Sail's docker-compose.yml file to the root of your application
php artisan sail:install
How to run your Laravel app using Sail?
Once all required installations have been done, we can now use sail in Laravel app.
Remember that your services run on different docker containers. Therefore cannot run Laravel commands directly from your terminal. Hence, use a Sail Command Line Interface.
This new CLI enables you to run commands inside a running container without logging in to container using SSH.
# Run Laravel Project Locally
# Following command will run all containers
# Defined in docker-compose.yml file
./vendor/bin/sail up
# If you want to stop all running containers
./vendor/bin/sail stop
# To check what containers are running run
# It will list all containers if any of them running
./vendor/bin/sail
# To destroy your running laravel app run
# This command will destroy all of your docker containers
./vendor/bin/sail down
# If you want to run unit tests
./vendor/bin/sail test
./vendor/bin/sail test --group orders
You can also run your artisan commands or other commands in Laravel Sail, as shown below.
# run artisan command using sail
# use following syntax for your artisan commands
./vendor/bin/sail artisan <command>
# example
./vendor/bin/sail artisan queue:work
# if you want to execute php command or script use
# following syntax for php commands
./vendor/bin/sail php <command>
# example
./vendor/bin/sail php --version
# to run composer commands using sail
# use following syntax
./vendor/bin/sail composer <command>
# exaamples
./vendor/bin/sail composer install
./vendor/bin/sail composer update
./vendor/bin/sail composer require laravel/sanctum
# if your project runs on npm or node or yarn use
# following sytaxes
./vendor/bin/sail npm <command>
./vendor/bin/sail yarn <command>
./vendor/bin/sail node <command>
# examples
./vendor/bin/sail yarn install
./vendor/bin/sail npm install
./vendor/bin/sail node --version
# if you want to ssh into container
./vendor/bin/sail bash
# if you want to check container logs
./vendor/bin/sail logs -f
# if you want to restart specific service
./vendor/bin/sail restart <service_name>
./vendor/bin/sail restart mysql
Hope you liked this tutorial. Stay tuned for my upcoming articles on Laravel!