A web application has different environment variables. For example, the Development or Local, then Staging, and finally Production. You may also have various developer working on a single project. So, environment variables should be set for each. The file that will define your Laravel application’s environment variables is the Laravel env file.
Note: You cannot commit a .env to your git project.
The default .env file has common configuration values which may differ depending whether an application runs globally or locally, or on the production server. For example, the .env file holds database credentials, which might be different for the database on your local computer and on the production server.
The .env.example file can hold placeholder keys that allows different developers to use different values as per their need.
You should not add sensitive information in either the .env or .env.example file that can create security issues and make hackers sniff data from your repository.
Consider following diagram:
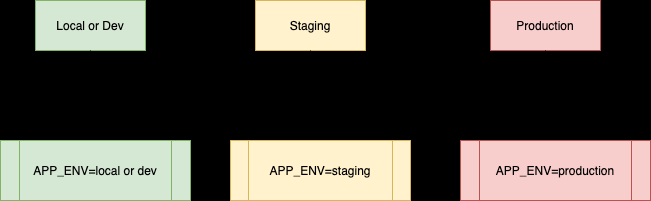
A Laravel Application has different environments as shown above. All will have a separate .env file. As while moving the app from one server to another, we never commit the .env file.
We can set our APP_ENV variable on each environment with different values. Say you can run a specific logic when env is either in staging or local. You may use following Laravel function to check the environment.
if (App::environment('prduction')) {
// The environment is production
// Run your production env specific logic here
}
if (App::environment(['local', 'staging'])) {
// The environment is either local OR staging...
// Run your ocal OR staging env specific logic here
}
How to fetch specific key from .env file in Laravel 8?
Suppose you have following keys/values defined in your .env file:
DB_PORT=3306
DB_HOST=mysql
DB_USERNAME=sail
DB_CONNECTION=mysql
DB_PASSWORD=password
DB_DATABASE=example_app
If you want to use one of the above key in your controller or any other classes, you may use following function to fetch the variable’s value.
# print the value of DB_HOST
echo env('DB_HOST', false);
You can call the env function and pass the variable defined inside it. The second argument is the default value of any variable. This will be returned if no environment variable exists for a given key.