We need to first understand the importance of Laravel, Why it is popular and why we should choose it for our web project? Below we have listed the amazing benefits that Laravel Framework offers, these would surely compel you to use the framework for your next web development project!
- Simple to understand and use
- Very Secure
- Improved Website performance
- Greatly handles high-traffic on website
- Used by more than 1.1 million websites worldwide
- Open source with wide community support
- Laravel Framework Features:
- MVC (Model-View-Controller) Structure
- Object Oriented
- Blade Template Engines for Views
- Eloquent ORM for Database Interactions
- In-build Authentication
- Automated Unit Testing
- Automated Task Scheduling
How to start as beginner?
There is always some difficulty in learning new things. however, Laravel is an ease-to-use and understand framework. In this tutorial, we will walk through the basic concepts and fundamental building blocks in Laravel, required to create any web project.
Main Learning Points:
- How to install Laravel?
- Understand the MVC Structure
- Create first page with Laravel
How to install Laravel Framework?
We assume you got a new computer with no packages installed. So, we will begin from fresh installation of everything.
Firstly, you need to install Docker, so find the link below to download and install it on your machine.
Download Docker here
Why we need Docker?
Suppose you need to create a blog website. So, here are some perquisites you need to install to run your blog website.
- PHP – Software Language
- Laravel Framework – MVC Structure like Laravel that depends on PHP Language
- Server – a web server like Apache or Nginx to run your website
- Database – to store your blog data, e.g. MySQL
We need all of the above in our local machine. However, it is hard to manage varying versions of each of the listed libraries. So, Docker helps us create virtual containers to install all of he above dependencies.
Later, we can scrap these containers using a single command, when we don’t need the above libraries. Suppose our blog site needs following libraries.
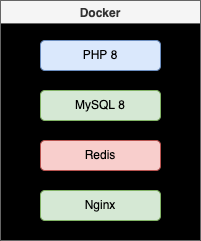
We can install all of the above using a Laravel tool known as Sail. It is a Laravel Command Line utility based on Docker, that allows us to install and manage various libraries and tools required for our Laravel project.
So, next click the link below to install Laravel Project using Sail.
Install Sail here.
We hope you understand well about Docker and Sail by now, and have installed Laravel framework using sail commands as mentioned above.
Understanding MVC concept
The next step is to understand the basic concept of MVC. It stands for Model, View, and Controller. You need to first understand how the request/response cycle works.
Consider the diagram below.
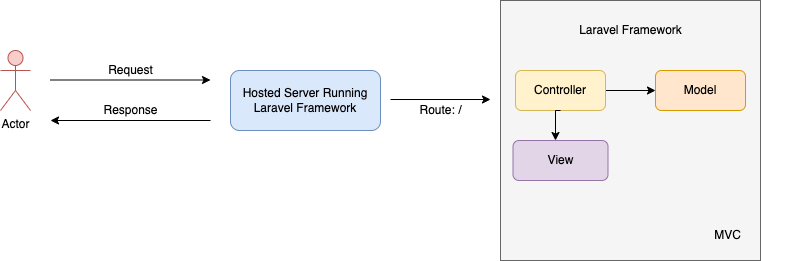
When a user goes through a website, a request is made via browser to the remote server, where the Laravel application is running. The remote server responds with some data as a response.
We will handle how Laravel handles requests and responses. Suppose a user types the URL: https://scratchcoding.dev to web browse like chrome. The diagram below shows how the request travels in the Laravel framework.

The Laravel framework will first pass the URL using a router. Each laravel route is tied to a specific controller method. Laravel finds the route and creates a request object to send the request to Laravel Controller.
Laravel Controllers are basically controller classes with various methods tied to specific routes. Once a request goes to the controller method, it can perform various tasks as listed below.
- Takes the requestobject
- Calls a model class and interacts with the database
- Sends data to a view, which is a blade template file containing HTML
- View parses all data to proper locations and returns back to the Controller
- Controller creates a response class to send requests back to the user and display on View
That’s how the Laravel MVC structure works!
Let us now go through a practical example to make you better understand these concepts.
Create first page using Laravel
We will now create a sample page to better understand the MVC structure of Laravel. A route is basically a URL we type in the browser, that is linked to a specific controller method.
Suppose if a user types https://scratchcoding.dev and a welcome message should come up. We should first create a route for this in our routes directory.
As the app users are using a web browser, so all routes go under the web.php file inside our routes directory. So, use your favourite editor and define your first route as listed below.
use App\Http\Controllers\HomeController;
Route::get('/', [HomeController::class, 'index']);
When we define the above route in routes/web.php, we are telling Laravel that whenever a user visits the ‘/’ URL appended to your blog website, in the browser, a request will be sent to the HomeController@index
method in Controller class.
So, next we will create a controller, named as HomeController
, to write the index
method. So, open your terminal, and type in the command given below.
# go to your laravel project directory
cd example-app
# create a new controller
./vendor/bin/sail artisan make:controller HomeController
The command above will create a controller file names as HomeController
in your app/Http/Controllers directory.
Now open the HomeController
file, and write the index method with the code below.
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
class HomeController extends Controller
{
public function index()
{
// you can call some model here
// store data in a variable and
// pass this data to your view file
// passing data to view file
return view('home/index', [
"message" => "Welcome to Scratch Coding"
]);
}
}
Next, you will create your view file, as index.blade.php
in your resources/views/home directory. So, open your resources/views/home/index.blade.php file in your code editor and write in the code below.
<h1>{{ $message }}</h1>
By now, we have done the following steps:
- Define route that points to a specific controller method. i.e. HomeController@index
- Define controller with the index method
- Define view file that is called from the controller index method
You may now go to your browser and type http://localhost/ to see your ‘Welcome to Scratch Coding‘ message.
If you are a beginner to the Laravel framework, you should practice the things given below to improve your skills and rise to level of intermediate or advance Laravel developer.
Intermediate Developer
- Practice small application on how to create different routes, models, controllers and views
- understand how many different ways you can create routes
- understand what methods and objects are available in controller class that you can use
- what things you can pass to your view file and how you can parse this data
- how to create models and how to use them in controller classes
- what is env and config files?
- Laravel Sail
- Form Validation
- request/response cycle
- Laravel Views
Advanced Developer
- learn about middlewares
- error handling
- Learn about artisan commands
- background jobs or queues
- localization
- package development
- modular concept
- Authentication based on roles
- asset management
- sessions
- caching techniques
- rate limiting
- notifications
- api/model resources
- Unit Testing
- Model Scopes
- View Composers
- build production docker image for Laravel
These may be a lot of concepts to learn and practice. However, regular practice can steadily make you a better developer. Go through my articles on Laravel Framework on this blog to sharpen your skills and learn advance concepts.
Laravel developers are very well paid, and you should have the right knowledge about the Laravel framework and associated concepts. Feel free to message us via the contact form if you have any concerns or need explanations for the next steps in your application development.
Share our articles with your friends! Happy Coding!